TL;DR: Flash new software to a cheap STLink v2 clone to get an integrated gdb
Essentially, I just followed the the instructions in their wiki. I still had some problems guessing the pinout and documented my progress.
Hardware:
To program the controller with new software you need a second programmer. I used another STLink clone to flash the new software. GND and VDD is easy, as it is led out on the programming header. Just connect this to the target.
For SWDIO and SWCLK you have to look at the datasheet. Which says, that PA14 is SWCLK and PA13 is SWDIO. Head over to the used package and locate those pins:
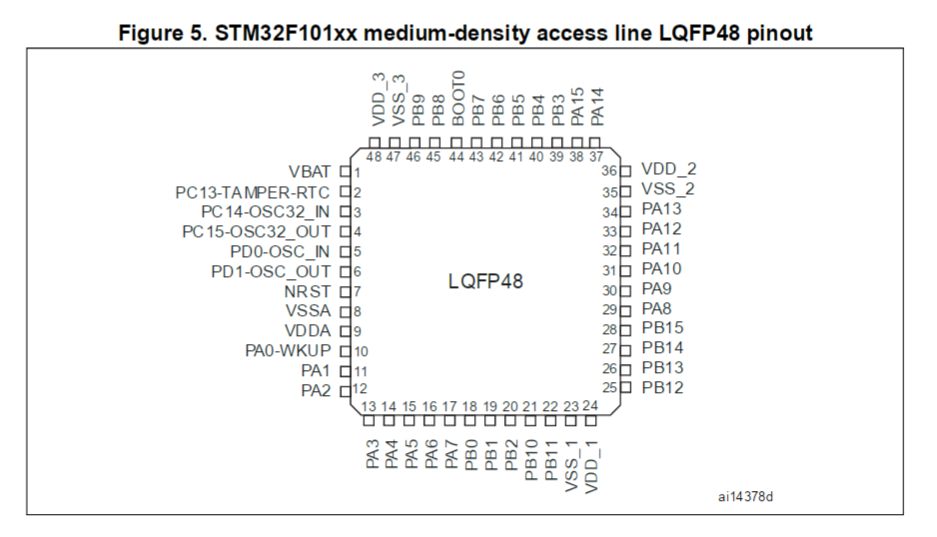
source: STM32F101x8 Datasheet Rev 17 DocID13586 from st.com
PA14 is the rightmost pin in the top line. This means our pinout from top to bottom is
GND – SWCLK – SWDIO – 5V
I’ve connected only those 2 pins using jumper cables and enameled copper wire. Remember that the bump on the package indicates top left. The imprint doesn’t matter.
Software:
The LED doesn’t work per default with my STLink hardware. So I circumvented the hardware detection and set it manually to STLink v2 in ./src/platforms/stlink/platform.c
This is the change:
uint16_t led_idle_run; /* Pins PC[14:13] are used to detect hardware revision. Read * 11 for STLink V1 e.g. on VL Discovery, tag as hwversion 0 * 10 for STLink V2 e.g. on F4 Discovery, tag as hwversion 1 */ int platform_hwversion(void) { static int hwversion = -1; int i; if (hwversion == -1) { gpio_set_mode(GPIOC, GPIO_MODE_INPUT, GPIO_CNF_INPUT_PULL_UPDOWN, GPIO14 | GPIO13); gpio_set(GPIOC, GPIO14 | GPIO13); for (i = 0; i<10; i++) hwversion = ~(gpio_get(GPIOC, GPIO14 | GPIO13) >> 13) & 3; // FIXME: hardware is set to STLink v2 manually hwversion = 1; switch (hwversion) { case 0: led_idle_run = GPIO8; break; default: led_idle_run = GPIO9; } } return hwversion; }
Build with
make PROBE_HOST=stlink
Flashing:
This is the output when flashing with another STLink v2:
while true; do openocd -f interface/stlink-v2.cfg -f target/stm32f1x_stlink.cfg -c "init" -c "halt" -c "stm32f1x unlock 0" -c "shutdown"; sleep 1; done
The while loop makes wiggling those flimsy cables trying to get a connection easier.
Open On-Chip Debugger 0.9.0 (2016-12-03-11:52) Licensed under GNU GPL v2 For bug reports, read http://openocd.org/doc/doxygen/bugs.html WARNING: target/stm32f1x_stlink.cfg is deprecated, please switch to target/stm32f1x.cfg Info : auto-selecting first available session transport "hla_swd". To override use 'transport select <transport>'. Info : The selected transport took over low-level target control. The results might differ compared to plain JTAG/SWD adapter speed: 1000 kHz adapter_nsrst_delay: 100 none separate Info : Unable to match requested speed 1000 kHz, using 950 kHz Info : Unable to match requested speed 1000 kHz, using 950 kHz Info : clock speed 950 kHz Info : STLINK v2 JTAG v17 API v2 SWIM v4 VID 0x0483 PID 0x3748 Info : using stlink api v2 Info : Target voltage: 3.234782 Info : stm32f1x.cpu: hardware has 6 breakpoints, 4 watchpoints Info : device id = 0x20036410 Info : flash size = 128kbytes target state: halted target halted due to breakpoint, current mode: Handler HardFault xPSR: 0x61000003 pc: 0x2000003a msp: 0xffffffdc stm32x unlocked. INFO: a reset or power cycle is required for the new settings to take effect. shutdown command invoked ^C
while true; do st-flash erase; sleep 1; done
st-flash 1.2.0-147-g3de5cf0 2016-12-03T13:54:02 INFO /home/avion/Documents/programming/embedded/stm32/stlink/src/usb.c: -- exit_dfu_mode 2016-12-03T13:54:02 INFO /home/avion/Documents/programming/embedded/stm32/stlink/src/common.c: Loading device parameters.... 2016-12-03T13:54:02 INFO /home/avion/Documents/programming/embedded/stm32/stlink/src/common.c: Device connected is: F1 Medium-density device, id 0x20036410 2016-12-03T13:54:02 INFO /home/avion/Documents/programming/embedded/stm32/stlink/src/common.c: SRAM size: 0x5000 bytes (20 KiB), Flash: 0x20000 bytes (128 KiB) in pages of 1024 bytes Mass erasing st-flash 1.2.0-147-g3de5cf0 2016-12-03T13:54:03 INFO /home/avion/Documents/programming/embedded/stm32/stlink/src/common.c: Loading device parameters.... 2016-12-03T13:54:03 INFO /home/avion/Documents/programming/embedded/stm32/stlink/src/common.c: Device connected is: F1 Medium-density device, id 0x20036410 2016-12-03T13:54:03 INFO /home/avion/Documents/programming/embedded/stm32/stlink/src/common.c: SRAM size: 0x5000 bytes (20 KiB), Flash: 0x20000 bytes (128 KiB) in pages of 1024 bytes Mass erasing ^C
st-flash --reset write blackmagic.bin 0x8002000
st-flash 1.2.0-147-g3de5cf0 2016-12-03T13:56:41 INFO /home/avion/Documents/programming/embedded/stm32/stlink/src/common.c: Loading device parameters.... 2016-12-03T13:56:41 INFO /home/avion/Documents/programming/embedded/stm32/stlink/src/common.c: Device connected is: F1 Medium-density device, id 0x20036410 2016-12-03T13:56:41 INFO /home/avion/Documents/programming/embedded/stm32/stlink/src/common.c: SRAM size: 0x5000 bytes (20 KiB), Flash: 0x20000 bytes (128 KiB) in pages of 1024 bytes 2016-12-03T13:56:41 INFO /home/avion/Documents/programming/embedded/stm32/stlink/src/common.c: Attempting to write 54724 (0xd5c4) bytes to stm32 address: 134225920 (0x8002000) Flash page at addr: 0x0800f400 erased 2016-12-03T13:56:42 INFO /home/avion/Documents/programming/embedded/stm32/stlink/src/common.c: Finished erasing 54 pages of 1024 (0x400) bytes 2016-12-03T13:56:42 INFO /home/avion/Documents/programming/embedded/stm32/stlink/src/common.c: Starting Flash write for VL/F0/F3 core id 2016-12-03T13:56:42 INFO /home/avion/Documents/programming/embedded/stm32/stlink/src/flash_loader.c: Successfully loaded flash loader in sram 53/53 pages written 2016-12-03T13:56:45 INFO /home/avion/Documents/programming/embedded/stm32/stlink/src/common.c: Starting verification of write complete 2016-12-03T13:56:46 INFO /home/avion/Documents/programming/embedded/stm32/stlink/src/common.c: Flash written and verified! jolly good!
st-flash write blackmagic_dfu.bin 0x8000000
st-flash 1.2.0-147-g3de5cf0 2016-12-03T13:57:04 INFO /home/avion/Documents/programming/embedded/stm32/stlink/src/common.c: Loading device parameters.... 2016-12-03T13:57:04 INFO /home/avion/Documents/programming/embedded/stm32/stlink/src/common.c: Device connected is: F1 Medium-density device, id 0x20036410 2016-12-03T13:57:04 INFO /home/avion/Documents/programming/embedded/stm32/stlink/src/common.c: SRAM size: 0x5000 bytes (20 KiB), Flash: 0x20000 bytes (128 KiB) in pages of 1024 bytes 2016-12-03T13:57:04 INFO /home/avion/Documents/programming/embedded/stm32/stlink/src/common.c: Attempting to write 6732 (0x1a4c) bytes to stm32 address: 134217728 (0x8000000) Flash page at addr: 0x08001800 erased 2016-12-03T13:57:04 INFO /home/avion/Documents/programming/embedded/stm32/stlink/src/common.c: Finished erasing 7 pages of 1024 (0x400) bytes 2016-12-03T13:57:04 INFO /home/avion/Documents/programming/embedded/stm32/stlink/src/common.c: Starting Flash write for VL/F0/F3 core id 2016-12-03T13:57:04 INFO /home/avion/Documents/programming/embedded/stm32/stlink/src/flash_loader.c: Successfully loaded flash loader in sram 6/6 pages written 2016-12-03T13:57:04 INFO /home/avion/Documents/programming/embedded/stm32/stlink/src/common.c: Starting verification of write complete 2016-12-03T13:57:04 INFO /home/avion/Documents/programming/embedded/stm32/stlink/src/common.c: Flash written and verified! jolly good!
Conclusion:
That was fast, easy and useful.